Deeper Dive into Routes and JSON API
-
meFqulted
- January 13, 2024
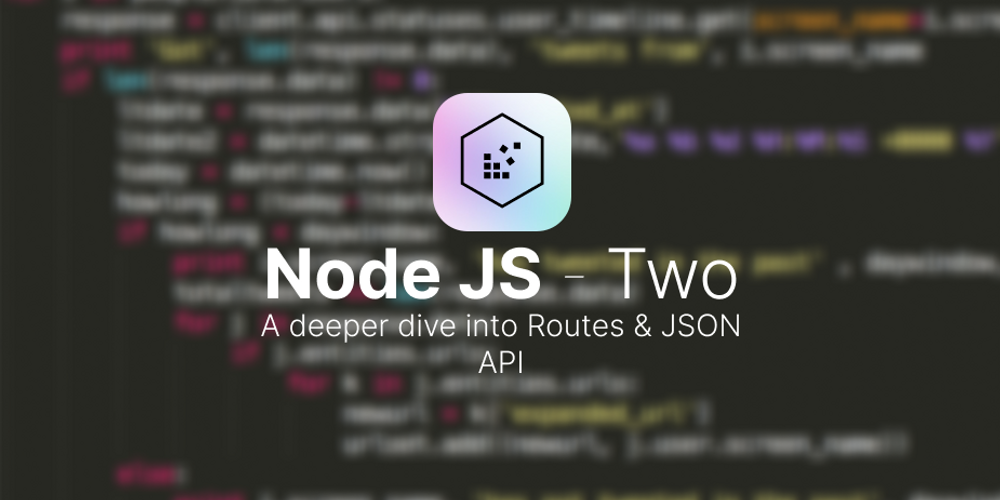
A deeper dive into Routes & JSON API
Let’s dive deeper into Express by exploring various HTTP methods (GET, POST, UPDATE, DELETE, PATCH) and creating JSON API endpoints with sample data.
Installing Required Modules
Before we start, make sure you have Express installed. If not, install it using:
npm install express
Setting Up Express Application
Create a new file, let’s call it app.js
, and set up the basic Express application:
const express = require('express');
const app = express();
app.use(express.json());
// Basic route
app.get('/', (req, res) => {
res.send('Welcome to our Express API!');
});
// Start the server
app.listen(3000, () => {
console.log(`Server running at http://localhost:3000/`);
});
Creating JSON API Endpoints
1. GET Route with Sample Data
const express = require('express');
const app = express();
app.use(express.json());
// Sample data
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' },
];
// GET route to fetch all users
app.get('/users', (req, res) => {
res.json(users);
});
// Start the server
app.listen(3000, () => {
console.log(`Server running at http://localhost:3000/`);
});
Now, visiting http://localhost:3000/users
should return a JSON array with user data.
2. POST Route to Add Data
// (previous code)
// POST route to add a new user
app.post('/users', (req, res) => {
const newUser = req.body;
users.push(newUser);
res.json(newUser);
});
// (rest of the code)
Use tools like Postman to send POST requests to http://localhost:3000/users
with JSON data to add new users.
3. UPDATE (PUT) Route
// (previous code)
// PUT route to update user by ID
app.put('/users/:id', (req, res) => {
const { id } = req.params;
const updatedUser = req.body;
users.forEach((user, index) => {
if (user.id == id) {
users[index] = { ...user, ...updatedUser };
res.json(users[index]);
}
});
res.status(404).json({ message: 'User not found' });
});
// (rest of the code)
Send PUT requests to http://localhost:3000/users/1
with updated user data to modify an existing user.
4. DELETE Route
// (previous code)
// DELETE route to remove user by ID
app.delete('/users/:id', (req, res) => {
const { id } = req.params;
const index = users.findIndex((user) => user.id == id);
if (index !== -1) {
const deletedUser = users.splice(index, 1)[0];
res.json(deletedUser);
} else {
res.status(404).json({ message: 'User not found' });
}
});
// (rest of the code)
Send DELETE requests to http://localhost:3000/users/1
to remove a user by ID.
5. PATCH Route
// (previous code)
// PATCH route to partially update user by ID
app.patch('/users/:id', (req, res) => {
const { id } = req.params;
const partialUpdate = req.body;
users.forEach((user, index) => {
if (user.id == id) {
users[index] = { ...user, ...partialUpdate };
res.json(users[index]);
}
});
res.status(404).json({ message: 'User not found' });
});
// (rest of the code)
Send PATCH requests to http://localhost:3000/users/1
with partial user data to update specific fields.
This lesson covers basic CRUD operations using Express with various HTTP methods. Feel free to experiment and explore more features as you continue building your Express applications!